Julia Data Structures
Julia Data Structures
The following are some of the most common data structures we end up using when performing data analysis on Julia:
- Vector(Array) – A vector is a 1-Dimensional array. A vector can be created by simply writing numbers separated by a comma in square brackets. If you add a semicolon, it will change the row. Vectors are widely used in linear algebra.
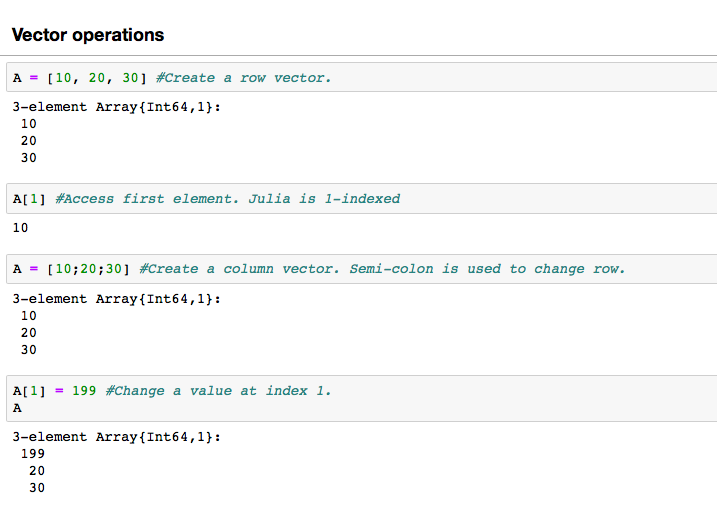
Note that in Julia the indexing starts from 1, so if you want to access the first element of an array you’ll do A[1].
- Matrix – Another data structure that is widely used in linear algebra, it can be thought of as a multidimensional array. Here are some basic operations that can be performed in a matrix
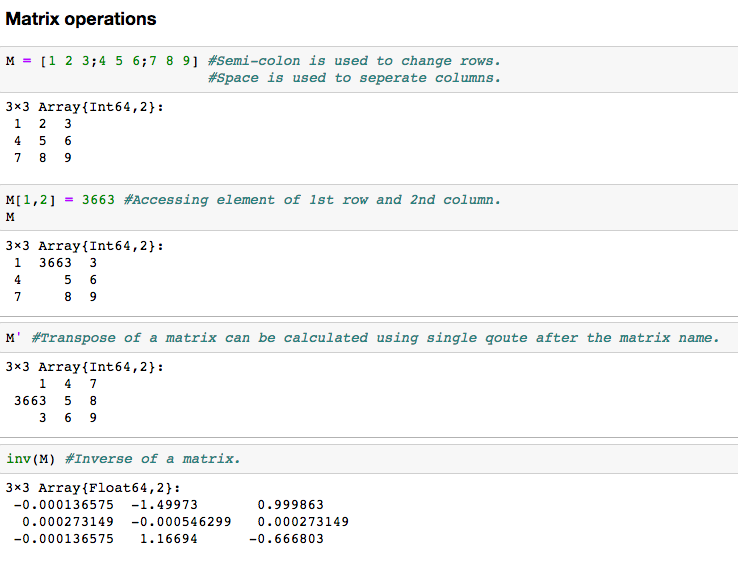
- Dictionary – Dictionary is an unordered set of key: value pairs, with the requirement that the keys are unique (within one dictionary). You can create a dictionary using the Dict() function.
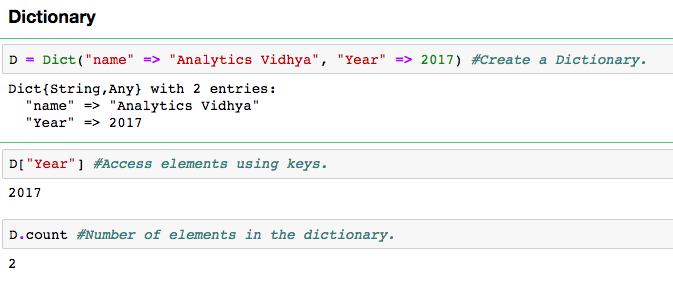
Notice that “=>” operator is used to link key with their respective values. You access the values of the dictionary using its key.
- String – Strings can simply be defined by use of double ( ” ) or triple ( ”’ ) inverted commas. Like Python, strings in Julia are also immutable(they can’t be changed once created). Look at the error below.
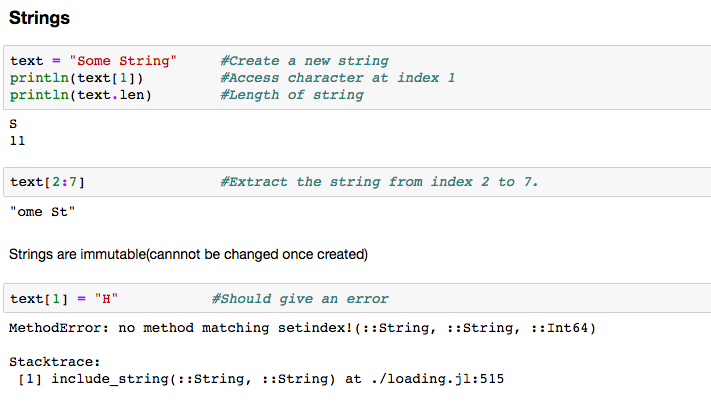
Loops, Conditionals in Julia
Like most languages, Julia also has a FOR-loop which is the most widely used method for iteration. It has a simple syntax:
for i in [Julia Iterable] expression(i) end
Here “Julia Iterable” can be a vector, string or other advanced data structures which we will explore in later sections. Let’s take a look at a simple example, determining the factorial of a number ‘n’.
fact=1 for i in range(1,5) fact = fact*i end print(fact)
Julia also supports the while loop and various conditionals like if, if/else, for selecting a bunch of statements over another based on the outcome of the condition. Here is an example,
if N>=0 print("N is positive") else print("N is negative") end
The above code snippet performs a check on N and prints whether it is a positive or a negative number. Note that Julia is not indentation sensitive like Python but it is a good practice to indent your code that’s why you’ll find code samples in this article well indented. Here is a list of Julia conditional constructs compared to their counterparts in MATLAB and Python.
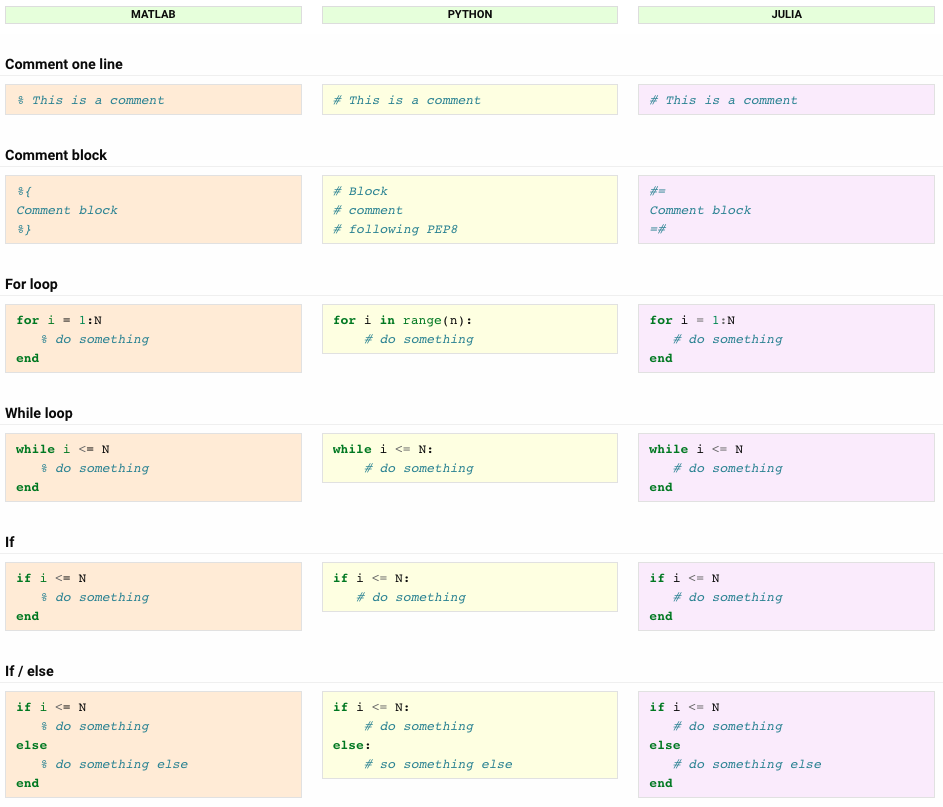
Thank you for sharing such a useful article. I had a great time. This article was fantastic to read. Continue to publish more articles on
ReplyDeleteAI Services
Data Engineering Services
Data Analytics Solutions
Data Modernization Solutions